Hardware issues, electronic components, schemas, Arduino, STM32, Robots, Sensors
-
Administrator
- Site Admin
- Posts: 81
- Joined: 26-Feb-2014, 17:54
Post
by Administrator » 11-Jul-2023, 22:08
Introduction to the Cortex-M7 MPU and AN4838 (Introduction to memory protection unit management on STM32 MCUs) says:
The MPU is an optional component for the memory protection. Including the MPU in the STM32 microcontrollers (MCUs) makes
them more robust and reliable. If the MPU is not enabled, there is no change in the memory system behavior.
When DMA is used in the project,
RAM memory used by DMA should not be cacheable. Is cache is used with DMA, cache need to be invalidated before each transaction.
Safe-Background MPU region 0 initialization:
Code: Select all
MPU_InitStruct.Enable = MPU_REGION_ENABLE;
MPU_InitStruct.Number = MPU_REGION_NUMBER0;
MPU_InitStruct.BaseAddress = 0;
MPU_InitStruct.Size = MPU_REGION_SIZE_4GB;
MPU_InitStruct.SubRegionDisable = 0x87;
MPU_InitStruct.TypeExtField = MPU_TEX_LEVEL0;
MPU_InitStruct.AccessPermission = MPU_REGION_NO_ACCESS;
MPU_InitStruct.DisableExec = MPU_INSTRUCTION_ACCESS_DISABLE;
MPU_InitStruct.IsShareable = MPU_ACCESS_SHAREABLE;
MPU_InitStruct.IsCacheable = MPU_ACCESS_NOT_CACHEABLE;
MPU_InitStruct.IsBufferable = MPU_ACCESS_NOT_BUFFERABLE;
HAL_MPU_ConfigRegion(&MPU_InitStruct);
[…other regions…]
// Enable the MPU, use default memory access for regions not defined here
HAL_MPU_Enable(MPU_PRIVILEGED_DEFAULT);
MPU_REGION_NO_ACCESS removes program access permissions (no read or write permissions, in both Handler and Thread modes).
MPU_INSTRUCTION_ACCESS_DISABLE blocks code execution.
TEX=0, C=0, B=0 would make this region Strongly Ordered, which is always shareable, so MPU_ACCESS_SHAREABLE has no effect, but also does not cause confusion by claiming the opposite while being ignored.
Existing code for other regions can be modified by incrementing the Region number as long as the total number of 8 (or 16, depending on device) regions is not exceeded.
Read more...
Video tutorial:
https://www.youtube.com/watch?v=QnDenqvzwyE
-
Administrator
- Site Admin
- Posts: 81
- Joined: 26-Feb-2014, 17:54
Post
by Administrator » 11-Jul-2023, 22:16
MPU subregions security for STM32
https://stackoverflow.com/questions/734 ... or-stm32h7
- Regions of 256 bytes or more are divided into 8 equal-sized subregions.
- Setting corresponding subregion bit excludes subregion from MPU region.
The subregion option is activated which means, it will be divided into 8 subregions starting from 0x0. Subregion size is 4GB/8 = 512MB = 0x2000 0000 bytes. According to Cortex-M7 memory map:
And SRD is set to
0x87 = 1000 0111b which means the MPU will be enabled only on (blue lines):
- On-chip peripheral address space???,
- External RAM,
- Shared device space.
One background region is created during initialization. This region is the only active one for privileged code. Module specific regions are configured every time there is a task switch into user code.
More information here:
https://developer.arm.com/documentation ... it?lang=en
-
Attachments
-
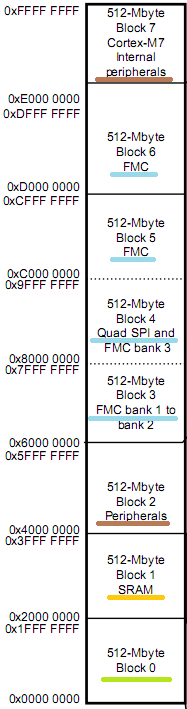
- CortexM7-MPU.png (24.49 KiB) Viewed 6509 times
-
Administrator
- Site Admin
- Posts: 81
- Joined: 26-Feb-2014, 17:54
Post
by Administrator » 13-Jul-2023, 22:44
Example for UART DMA TX & RX.
uint8_t tx_buffer[512] @ 0x20020000;
uint8_t rx_buffer[512] @ 0x20020200;
Code: Select all
MPU_InitStruct.Enable = MPU_REGION_ENABLE;
MPU_InitStruct.Number = MPU_REGION_NUMBER1;
MPU_InitStruct.BaseAddress = 0x20020000;
MPU_InitStruct.Size = MPU_REGION_SIZE_1KB;
MPU_InitStruct.SubRegionDisable = 0x0;
MPU_InitStruct.TypeExtField = MPU_TEX_LEVEL1;
MPU_InitStruct.AccessPermission = MPU_REGION_FULL_ACCESS;
MPU_InitStruct.DisableExec = MPU_INSTRUCTION_ACCESS_DISABLE;
MPU_InitStruct.IsShareable = MPU_ACCESS_SHAREABLE;
MPU_InitStruct.IsCacheable = MPU_ACCESS_NOT_CACHEABLE;
MPU_InitStruct.IsBufferable = MPU_ACCESS_NOT_BUFFERABLE;